Simple Player Movement
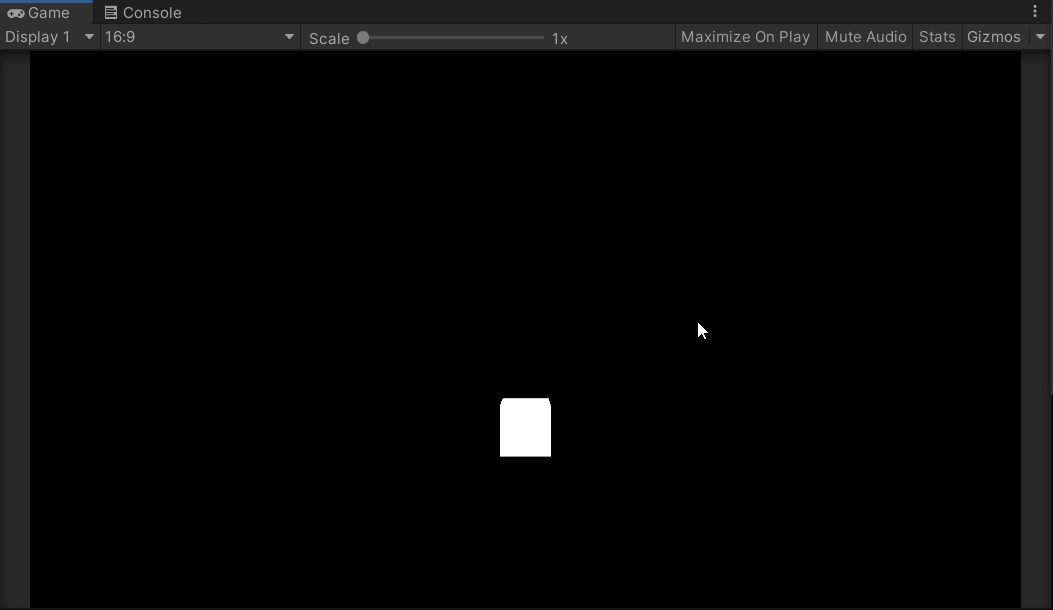
Good afternoon!
Today we are going to quickly look at setting up player movement. Now, there are a few ways to move an object, but for now we are just going to look at moving it by using its transform component.
Moving an object by its transform is just like grabbing that object and dragging it. It is generally how you would move an object in the scene view to adjust its placement by grabbing one of the three axes and dragging it. And to do it at runtime based off player input is easy to set up, just two steps and four lines of code.
Step 1: Create Script
Create a C# and give it a name (Player for example). Then attach that script to your player object and open it up.
Step 2: Write that Code
So to detect player input, we are going to use the axes already created with Unity. Navigate to Project Settings>Input Manager and it will give you the list of axes. We are going to use the horizontal and vertical axes to detect player input.

As you can see above, the name of the horizontal axis is, shockingly, “Horizontal”, and it is already mapped to the left and right arrow keys and the a and d keys. Just below it, you can see the vertical axis, and it is mapped to the up and down arrow keys and the w and s keys. The return type for both these axes is a float, between -1 and 1.
Now let’s pop over to the script and start coding.
To give a cleaner look to our code, I create three local variable to use in the translation of the player: two floats and a Vector3. The floats are for the horizontal and vertical inputs, and the Vector3 is the direction that the player will be moving.
So to get a reference to the axes, we use Input.GetAxis(“Axis Name”) where the axis name is the name shown in the properties under the axis in the input manager. For the horizontal axis it is “Horizontal”, and for vertical, “Vertical”.
Then create a Vector3 for the player’s direction that has the horizontal input for the x component, the vertical input for the y component, and zero in this case for the z component. So for example, if you had the right arrow key pressed and nothing else, your horizontal input would be 1 and your vertical input would be zero. In that case, your player direction would be (1, 0, 0), one along the x axis and zero along both the y and z axes.

Next step is to apply the direction to the player’s transform component. This is done by calling transform.Translate() which takes in a Vector3. The transform.Translate changes the player’s x, y, and z position based on the Vector3 that we pass in. So in the above example, it would add 1 to the x component of the player’s transform and 0 to the y and z components.
The last step is to normalize the vector and apply a speed. We normalize it with Time.deltaTime which makes it frame rate independent. It we do not normalize it, then we’re modifying the player’s position each frame by the vector that we pass in, and since the Update method runs at about 60 fps, we’re changing the position 60 times per second.
Adding a speed variable, set to whatever speed you want, allows you to adjust the speed at which the player moves so he is not stuck at 1 mps. Since the direction vector is composed of the horizontal and vertical inputs, both of which have a max value of one, multiplying by a speed increases the distance covered by the player per second.
Hope it was helpful and see you next time!